How to get JavaScript to Interact with External Files and Data – Guest post by Liz Larsen
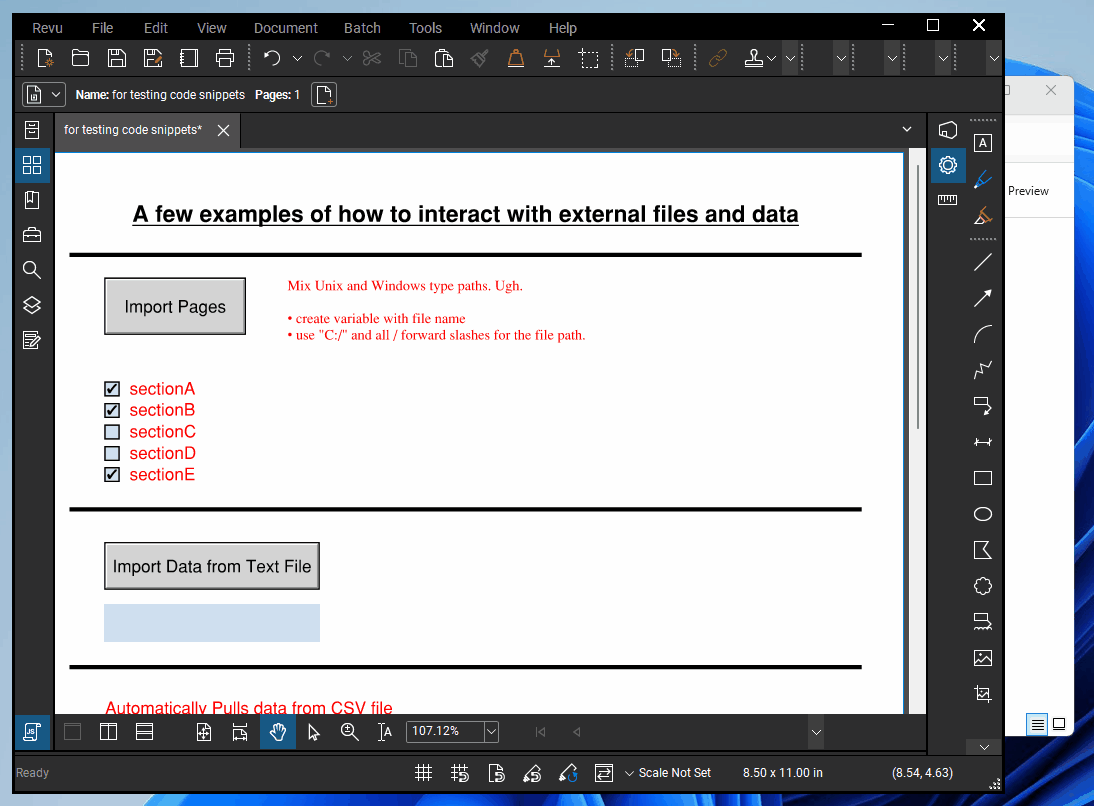
If you’ve spent any time at all in the Professional Learning Community we call the Bluebeam Brainery you’ve no doubt learned a thing or two from Liz Larsen. As a Structural Engineer, she works in the trenches and knows her stuff. She also has a gift for sharing in a understandable way. Continually pouring out value, you will find her on her Blog, YouTube channel, and several presentations throughout the industry. Liz was also in the Bluebeam spotlight on a recent blog post.
Keep an eye on the UChapter2 events page for her upcoming presentation.
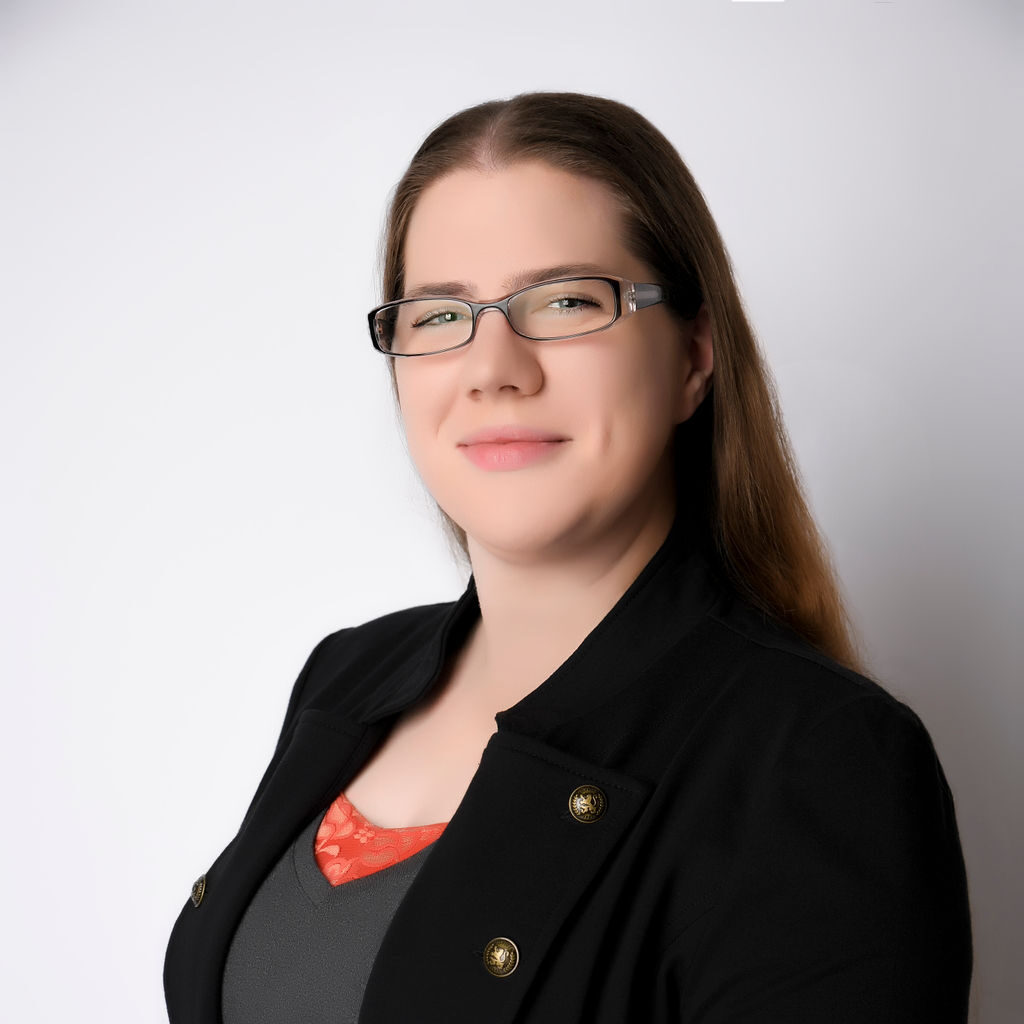
Liz is a structural engineer with a passion for technology. A Bluebeam user since 2015, Liz loves finding new ways to use Bluebeam and sharing her knowledge with others. In addition to multiple presentations with coworkers and clients, she is also a two time presenter at Bluebeam’s annual conference, XCON. Originally from upstate NY, Liz currently resides in Raleigh, North Carolina where she works for Stewart, a multi-disciplinary Civil Engineering firm.
Thank you Liz for all the wisdom and effort you put into this article to ensure the maximum value to readers. You’re well-known in the Bluebeam community and I speak for everyone when I say we appreciate everything you share. We are always ready to soak up the wisdom you’ve shared in this article, the activity in forums, your presentations throughout the industry, and your presence on LinkedIn and other channels.
I always leave these guest posts in the writer’s words without edit.
Liz Writes:
I think it’s a little overdue, but I finally sat down and compiled some of my methods to interact with external files and data using JavaScript in Bluebeam.
I’m going to throw a lot of information at you right now, so if you want to test it out, be sure to read everything to understand how works.
Some important information before we get into the nitty gritty:
- You must give Bluebeam permission to run JavaScript.
- Go to the Revu menu –> Preferences–> Advanced –> JavaScript. Then check Enable JavaScript and Add the “for testing code snippets.pdf” to the list of allowed files.
- Go to the Revu menu –> Preferences–> Advanced –> JavaScript. Then check Enable JavaScript and Add the “for testing code snippets.pdf” to the list of allowed files.
- You must keep the zip folder structure intact for the PDF to work.
- The file paths in the code are relative, meaning you can unzip this folder anywhere and it’ll work without the need to update the code for new file paths.
- Additionally, what this means is that the code still expects the external files to be in certain places relative to the PDF save location.
- Therefore, do not rearrange the files within the folder and expect them to still work. You can do this, but you’ll have to update the code.
- You’ll see a markup that reads: “Mix Unix and Windows type paths. Ugh.”
- This is how I talk to myself and I just didn’t bother removing the note from my past self to my future self.
- What I mean is:
- Windows file paths look something like: C:\data\myData.txt
- Unix file paths look something like: /c/data/myData.txt
- For the “Insert Pages” button, your file path will need to look like: C:/data/myData.txt
- Hopefully you can see it’s a bit of a mix between the two. This took me way too long to figure out. I don’t wanna talk about it.
The contents of the zip file are:
- PDF – “for testing code snippets.pdf”
- This is the PDF where the JavaScript lives.
- Let’s call this one the “main” PDF for simplicity.
- Folder – “Sections”
- This folder contains 4 PDFs for testing purposes.
- CSV file – “sample.csv”
- Contains a simple address book.
- JSON file – “sample.json”
- Contains some pricing data.
- Text file – “test.txt”
- Contains some sample text.
- Also exists to see how many times I can mix up “test” and “text” when writing the code and this blog post. Turns out the answer is “a lot”.
What does the “for testing code snippets” PDF actually do?
- It has a button called “Import Pages”:
- This can… insert pages.
- More specifically, it looks for external PDFs and inserts them at the end of the document.
- Even more specifically, it only inserts the PDFs that correspond to the checked check boxes.
- These external PDFs are in a subfolder called Sections.
- Currently, I’m lazy (all the time, actually, not just currently…) and I didn’t want to have to write more code, so I matched the names of the PDF files to the names of the check box form fields. Because of this, you can basically have any number of check boxes and matching PDFs without needing to update a single line of code, provided:
- The PDF name matches the check box form field name.
- The external PDFs are in a folder called “Sections” and that folder is saved in the same place as the “main” PDF.
- It’s clever, not lazy.
- I also added an error handler that will let you know if the JavaScript didn’t find the associated PDF.
- It has a button called “Import Data from Text File”:
- This can read text from a .txt file (named txt) and insert the contents into a form field text box.
- I didn’t do anything clever with this one. If you want to change the name of the text file, you’ll have to update the JavaScript.
- It has a dropdown form field:
- This can read data from a CSV file (named csv) and use that info to update a form field text box based on the dropdown selection.
- It has another dropdown form field:
- Same as previous bullet, but with a JSON file (named json).
- You can edit the JSON file by right clicking on the file and select Open With–> Notepad
- I included this for a couple reasons:
- I learned about this feature at XCON 2019 and wanted to include it.
- JSON, while not widely known outside of the coding community, is a common data format that you might find in various places throughout the internet and it’s nice to know how to extract information from it.
- According to ChatGPT:
JSON stands for JavaScript Object Notation. It is a standard text-based format for storing and transmitting data. JSON is popular with developers because it is human-readable, lightweight, and requires less coding. JSON files store data in key-value pairs and arrays. The keys serve as names and the values contain related data. JSON arrays are written inside square brackets. For example, “employees”:[{“firstName”:”John”, “lastName”:”Doe”}, {“firstName”:”Anna”, “lastName”:”Smith”},]. |
- An example I encountered in the real world is that the USGS has JSON formatted seismic data based on GPS coordinates.
“But, Liz,” I hear you ask, “How do I update the code?”
- First: have eXtreme or Complete
- Second: know/learn JavaScript
- For the buttons:
- Go into the Forms panel, select the button, go to the properties panel and scroll to the bottom. Under Actions, double click on JavaScript, then click Edit.
- Go into the Forms panel, select the button, go to the properties panel and scroll to the bottom. Under Actions, double click on JavaScript, then click Edit.
- For the dropdowns:
- Use keyboard shortcut CTRL+SHIFT+Jor find it under Tools –> Form –> JavaScript.
- Select the Dropdown Code and then click the pencil icon.
Additional important information
- All of the code uses the names of the form fields. In the JavaScript, look for getField(“formFieldName“), where formFieldNameis the exact name of the form field in the Forms It is case-sensitive.
- In the example above, if I were to add pdf to the Sections folder, the JavaScript would automatically find an insert the SectionE.pdf because of the way I’ve associated the two in the JavaScript.
- Currently, as shown, you will get a popup window telling you that SectionE does not exist.
- It pains me to say this, but it appears that having custom statuses in the documents you want to import breaks the code. Bluebeam freezes and you have to use the Task Manager to force close it.
- For example, I have PDF file SectionA. I open SectionA, add a text box, and Bluebeam automatically attaches my custom statuses from my profile into the SectionA Then later, when I’m trying to import SectionA using JavaScript, the whole thing crashes.
- This was not previously an issue with Revu 18 and earlier. I know because Bluebeam had to give me a temporary license to 18 when I presented at XCON because none of us could figure out why my PDF suddenly wasn’t working in 19.
- Throughout my code, you’ll see println()or app.alert(). These two tools are how I debug my code. When something isn’t working, I either print to console or I create a popup box to show me what my code is doing.
- For example, if I’m trying to insert an external file, and Bluebeam gives me an error, I’ll use println(filepath)to view what the filepath variable is at that point in code.
- When I’m done using that particular debugging tool, I’ll make it a comment by adding // in front of it so the code ignores it the next time it runs. This makes it easy to use it again when I need it. I just have to delete the // and update the output to whatever I want to see.
This was a lot of info, I know. But, as always, feel free to reach out to me on LinkedIn or UChapter2 and I’ll do my best to help you.
I hope you enjoyed Liz Larsen’s JavaScrpt value bombs. With Bluebeam Revu so versatile and customizable, you’re really only limited by your own creativity to develop powerful workflows. If Revu is changing the way you do business, I’d love to help tell your story here on UChapter2. Even the shortest stories can spark ideas. Email your story to me at Troy.DeGroot@UChapter2.com
Responses